HLS Player
The HMSHLSPlayer
component is an HLS player offered by 100ms that can be used to play HLS streams.
The player can take a URL to play the stream. It also sends analytics events related to the HLS
playback to the 100ms dashboard.
Requirements
- SDK version 1.7.0 or higher
How to Start HLS
-
Take a look at the HLS Streaming docs about how to start HLS stream in a rRoom.
-
Here's how to read the HLS URL from the room.
From the above links, we now know that we can get the HLS stream URL from ON_JOIN
and ON_ROOM_UPDATE
events.
How to Play an HLS Stream
Import HMSHLSPlayer component
import { HMSHLSPlayer } from '@100mslive/react-native-hms';
Play HLS Stream on HMSHLSPlayer component
-
Play the HLS Stream as soon as
HMSHLSPlayer
component is visible on screenWe can render the
HMSHLSPlayer
component and pass the HLS Stream URL to it to play the HLS stream.const streamURL = room.hlsStreamingState?.variants?.get(0)?.hlsStreamUrl; ...
<HMSHLSPlayer url={streamURL} style={{ width: 300, height: 600 }} />The
url
prop of HMSHLSPlayer component is optional, HMSHLSPlayer can automatically get the HLS stream URL from the HMSRoom if you render it when HLS stream has started -// --- snip --- const isHLSStreamRunning = room.hlsStreamingState?.running; ... if (isHLSStreamRunning) {
return <HMSHLSPlayer style={{ width: 300, height: 600 }} />;} return <Text>Waiting for strem to start</Text>;Checkout 2nd point of How to Start HLS section to know how to check if HLS Stream is running or not.
-
Render
HMSHLSPlayer
component first and Play laterWe can also call
play
method available on ref ofHMSHLSPlayer
component to play the HLS Stream.play
method accepts the HLS stream URL as a first parameter. However, It's optional, if we don't pass it, thenHMSHLSPlayer
component tries to read URL from HMSRoom.// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} style={{ width: 300, height: 600 }} /> ... // Now, we can call `play` method on `hmsHlsPlayerRef` anytime
hmsHlsPlayerRef.current?.play();
Note: We also have to pass width
and height
in style
prop for HLSPlayer to be visible on screen
Take a look at how the sample app does this if not clear.
Styling HMSHLSPlayer Component
HMSHLSPlayer
component accepts style prop like a react-native View
, for HLS Player to be visible on screen we have to give it some width
and height
.
<HMSHLSPlayer url={streamURL}
style={{ width: 300, height: 600 }}/>
You can also apply width and height on the basis of video stream's width and height, For this we have to get video streams resolution -
const resolution: { width: number; height: number; } | undefined = useHMSHLSPlayerResolution();
Note that resolution
will be undefined
initially, Therefore, You have to set static
width
and height
in start and use resolution
when it is not undefined
const resolution: { width: number; height: number; } | undefined = useHMSHLSPlayerResolution();
...<HMSHLSPlayer url={streamURL} style={{width: resolution ? resolution.width : 300,height: resolution ? resolution.height : 600,}} />
How to Stop the Playback
When the HMSHLSPlayer
component is unmounted, HLS stream playback is automatically stopped.
If you want to stop the stream playback without unmounting the HMSHLSPlayer
component, you can call stop
method available on the ref
of HMSHLSPlayer
component -
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... // Now, we can call `stop` method on `hmsHlsPlayerRef`
hmsHlsPlayerRef.current?.stop();
How to Show Video Player UI
By default, Video Player UI or Controls are hidden.
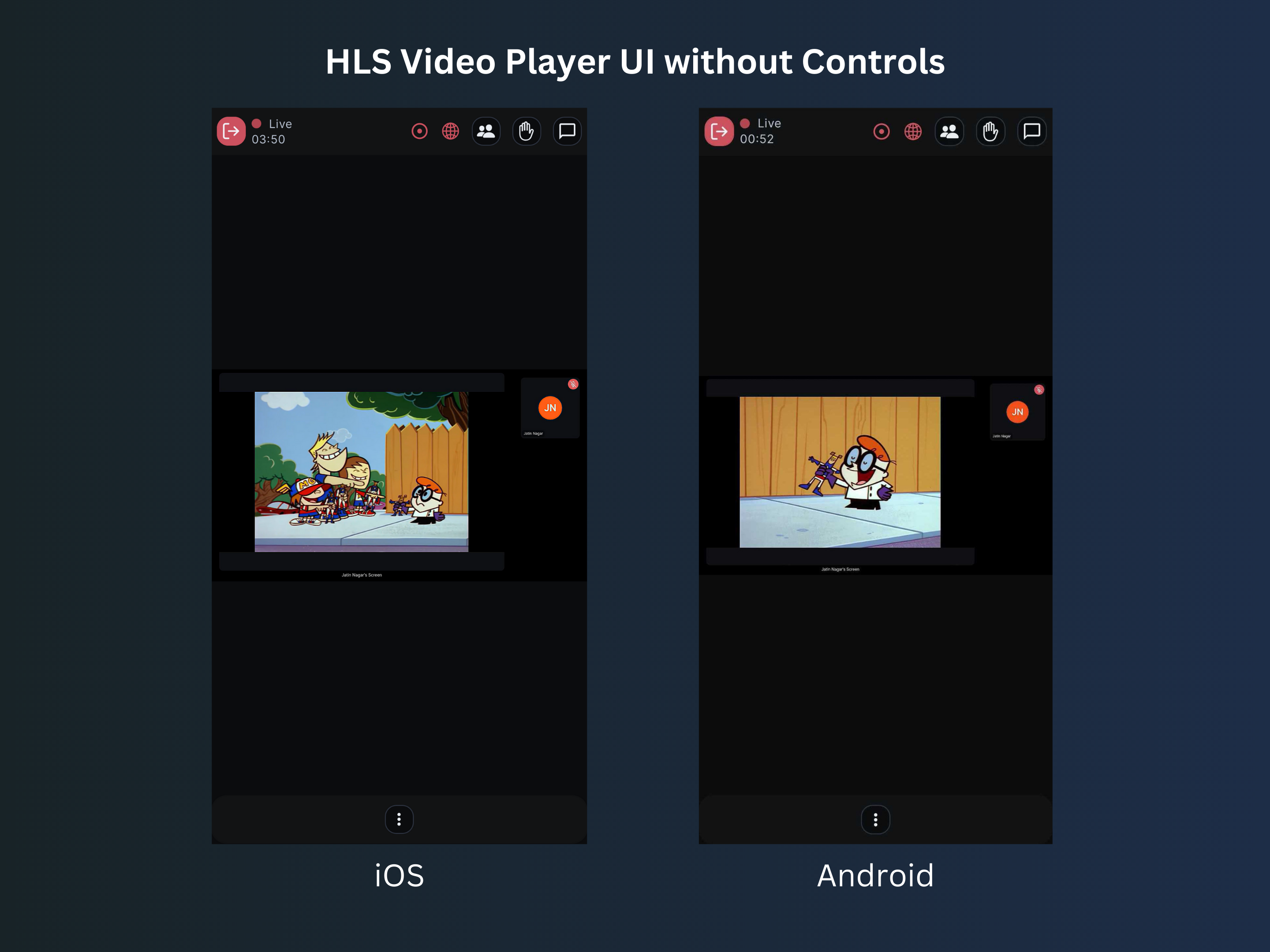
We can show Video Player UI or Controls by passing true
to enableControls
prop on HMSHLSPlayer
component -
<HMSHLSPlayer // Pass `true` to `enableControls` prop to show the Video Player Controls
enableControls={true}/>
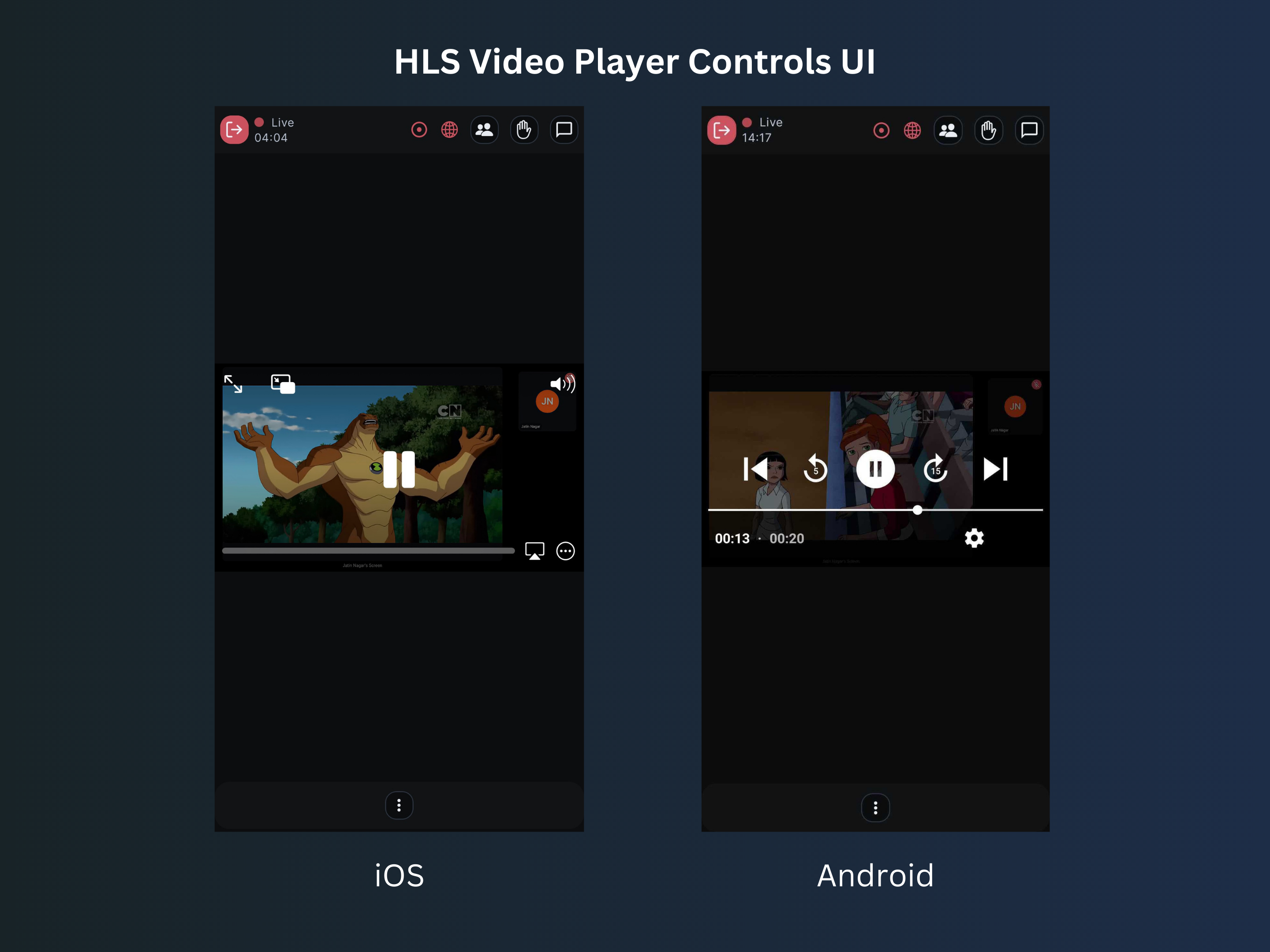
How to Pause and Resume the Playback
We can Pause and/or Resume the HLS Playback using the pause
and resume
methods respectively
available on the ref
of HMSHLSPlayer
component -
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign the above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ...
hmsHlsPlayerRef.current?.pause();hmsHlsPlayerRef.current?.resume();
How to Seek Forward or Backward
We can seek forward and/or backwards on the HLS Playback using the seekForward
and seekBackward
methods respectively
available on the ref
of HMSHLSPlayer
component.
seekForward
and seekBackward
methods accept number of seconds to seek by as a first parameter.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ...
// Seek Forward by 5 secondshmsHlsPlayerRef.current?.seekForward(5);// Seek Backward by 5 secondshmsHlsPlayerRef.current?.seekBackward(5);
How to Seek to Live Position
We can use seekToLivePosition
method available on the ref
of HMSHLSPlayer
component to go to the live position -
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... // Go to Live Position on HLS Stream
hmsHlsPlayerRef.current?.seekToLivePosition();
How to Change the Volume of HLS Playback
We can use setVolume
method available on the ref
of HMSHLSPlayer
component to change the playback volume levels.
setVolume
method accepts volume level as a first parameter, valid volume level is in [0-100]
range.
Volume is set to 100
level by default.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... hmsHlsPlayerRef.current?.setVolume(50); // use value from 0-100 range
How to enable or disable Closed Captions
Check If Closed Captions are supported in stream
We can use isClosedCaptionSupported
method available on the ref
of HMSHLSPlayer
component to check if captions are supported and available in the stream.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... const isSupported = await hmsHlsPlayerRef.current?.isClosedCaptionSupported();
Enable Closed Captions
We can use enableClosedCaption
method available on the ref
of HMSHLSPlayer
component to enable the captions in the stream.
Before enabling or disabling captions, you should check if captions are supported in the stream.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... await hmsHlsPlayerRef.current?.enableClosedCaption();
Disable Closed Captions
We can use disableClosedCaption
method available on the ref
of HMSHLSPlayer
component to disable the captions in the stream.
Before enabling or disabling captions, you should check if captions are supported in the stream.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... await hmsHlsPlayerRef.current?.disableClosedCaption();
Check If Closed Captions is enabled or not
We can use isClosedCaptionEnabled
method available on the ref
of HMSHLSPlayer
component to check if captions are enabled in stream or not.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... const isEnabled = await hmsHlsPlayerRef.current?.isClosedCaptionEnabled();
Show Closed Captions in UI
In case of iOS - iOS shows captions on its own. We can only enable, disable, check support or check enabled status in case of iOS.
In case of Android - Android also shows a default UI for captions. It is tied up with reset of the default controls of player, meaning captions only show up when you have enabled default controls of player. If you turn off default controls with enableControls = false
prop, captions will also be hidden.
However, Unlike iOS, Android gives us an option to render captions on UI ourselves, If captions are supported in stream, We can use useHMSHLSPlayerSubtitles
hook to get the live captions.
// Get live captions const captions = useHMSHLSPlayerSubtitles(); ... // Render captions <Text>{captions}</Text>
Note: If you are not getting captions, check if captions are supported and enabled.
How to get stream properties
We can use getPlayerDurationDetails
method available on the ref
of HMSHLSPlayer
component to get stream properties like duration, rolling window, etc.
// Create `ref` for `HMSHLSPlayer` component const hmsHlsPlayerRef = useRef(null); ... // Assign above created ref to `ref` prop of `HMSHLSPlayer` component <HMSHLSPlayer ref={hmsHlsPlayerRef} /> ... const { streamDuration, rollingWindowTime } = await hmsHlsPlayerRef.current?.getPlayerDurationDetails();
Note: In case of iOS, check the rollingWindowTime
property for getting the rolling window or duration of the stream. For android check the streamDuration
property.
How to Know the Stats Related to HLS Playback
Enable HLS Stats on the
By default, HMSHLSPlayer
component does not send playback stats. We can enable HLS Stats on
the HMSHLSPlayer
component using the enableStats
prop -
<HMSHLSPlayer // Pass `true` to `enableStats` prop to enable the HLS Player Stats
enableStats={true}/>
Use HLS Stats received from the
Now, we can use useHMSHLSPlayerStats
hook in any component to get the stats object -
// Step 1: Import `useHMSHLSPlayerStats` hook import { useHMSHLSPlayerStats } from '@100mslive/react-native-hms'; ... // Step 2: use `useHMSHLSPlayerStats` hook inside any Component const HLSStatsView = () => {
const { stats, error } = useHMSHLSPlayerStats();}
stats
object has the following interface -
interface HMSHLSPlayerStatsUpdateEventData { // current network speed of the user bandWidthEstimate: number; // total Bytes downloaded till this moment totalBytesLoaded: number; // An estimate of the total buffered duration from the current position bufferedDuration: number; // Distance of current playing position from live edge distanceFromLive: number; // total number of dropped frames droppedFrameCount: number; // bitrate of the current layer being played averageBitrate: number; // The height of the resolution of video videoHeight: number; // The width of the resolution of video videoWidth: number; };
An example of usage of useHMSHLSPlayerCue
hook is provided in the sample app in HLSPlayerStatsView Component.
How to Know When Player Changes State During Playback
The HLS player can be in one of the following states: playing
, stopped
, paused
, buffering
, failed
, or unknown
during playback.
We can use useHMSHLSPlayerPlaybackState
hook to know about the state change event during the playback -
// Step 1: Import `useHMSHLSPlayerPlaybackState` hook import { useHMSHLSPlayerPlaybackState } from '@100mslive/react-native-hms'; ... // Step 2: use `useHMSHLSPlayerPlaybackState` hook inside any Component const HLSView = () => {
const playbackState = useHMSHLSPlayerPlaybackState();}
HLS Player playback state can be one of variant of HMSHLSPlayerPlaybackState
enum -
enum HMSHLSPlayerPlaybackState { BUFFERING = 'buffering', FAILED = 'failed', PAUSED = 'paused', PLAYING = 'playing', STOPPED = 'stopped', UNKNOWN = 'unknown', }
How to Know When There is an Error During HLS Playback
We can use useHMSHLSPlayerPlaybackError
hook to know about the errors that happen during playback.
useHMSHLSPlayerPlaybackError
hook usage is just like the usage of useEffect
hook.
We pass a callback function to the useHMSHLSPlayerPlaybackError
hook as a first parameter and dependency array as a second parameter.
Whenever there is an error during playback, passed callback function will be called with error occurred.
useHMSHLSPlayerPlaybackError( // callback function (err) => { // logging playback error to console console.log(err); }, // dependency array [] );
HLS Player Playback Error has the following interface -
interface HLSPlayerPlackbackError { errorCode: string; errorCodeName: string; message?: string; };
How to Use HLS Timed Metadata with 100ms HLS player
When we use the 100ms HLS player to play the HLS stream, we can use useHMSHLSPlayerCue
hook to
get timed cue events when the player's current time matches that of a timed event in the HLS stream and use it's data to
show any UI like quizzes, poll etc. to HLS viewers.
useHMSHLSPlayerCue
hook usage is just like the usage of useEffect
hook.
We pass a callback function to the useHMSHLSPlayerCue
hook as a first parameter and dependency array as a second parameter.
Whenever there is a timed cue event, passed callback function will be called with cue data.
useHMSHLSPlayerCue( // callback function (cue) => { const payloadStr = cue.payloadval; if (typeof payloadStr === 'string') { // use the cue data payload as per your usecase like quizzes, polls and emoticons etc. } }, // dependency array [] );
HLS Player Cue data has the following interface -
interface HMSHLSPlayerPlaybackCue { // Unique id of the timed event id?: string; // String payload of the timed event payloadval?: string; // endDate of the timed event endDate?: Date; // startDate of the timed event startDate: Date; };
An example of usage of useHMSHLSPlayerCue
hook is provided in the sample app in HLSPlayerEmoticons Component.
How to Configure HLS Player to Send Analytics Events
There is no configuration needed, HMSHLSPlayer
component automatically sends Analytics Events to 100ms dashboard when the Room is active.
đź‘€ To see an example Android HLS Player implementation using 100ms SDK, checkout HLSView Component from our example project.